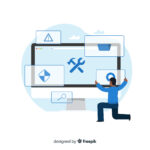
Must-Have WordPress Plugins for Your Website
August 9, 2024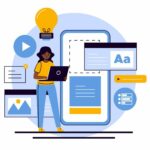
What Is A Page Builder? And Why You Should Use One
August 16, 2024Integrating a license manager API with your HISE audio plugin is essential for ensuring that only authorized users can access and use your software. In this guide, we’ll walk you through the steps to integrate the license manager API into your HISE project, including retrieving the JSON Web Token (JWT), fetching user licenses, and activating a license key.
Prerequisites
Before we begin, make sure you have the following:
- HISE Installed: Ensure you have the HISE software installed and set up on your development environment.
- License Manager API Access: Access to the license manager API endpoints.
- Basic Knowledge of JavaScript: Familiarity with JavaScript, as HISE uses JavaScript for scripting.
Step 1: Setting the Base URL
The first step is to configure the base URL of the license manager’s API. This URL will be used for all subsequent API requests.
Server.setBaseURL("https://www.yourdomain.com");
By setting the base URL, you ensure that all API calls are directed to the correct server.
Step 2: Retrieve the JSON Web Token (JWT)
The JSON Web Token (JWT) is necessary to authenticate API requests. You can obtain this token by calling the getJSONWebToken
function, which requires the user’s username and password.
inline function getJSONWebToken(username, password) {
payload = {
"username": username,
"password": password
};
Server.callWithPOST('/wp-json/jwt-auth/v1/token', payload, function(status, response) {
token = response.token;
Console.print("Token: " + token);
});
}
Store the token, username, and password on the user’s device encrypted for future API use.
Explanation:
- Endpoint: The
/wp-json/jwt-auth/v1/token
endpoint is called to generate a JWT token. - Payload: This contains the user’s
username
andpassword
. - Callback Function: After the API call, the token is stored in the
token
variable and printed to the console.
Step 3: Fetch User Licenses
Once the JWT token is retrieved, you can fetch all the license keys associated with the user. This is done through the getUserLicense
function.
inline function getUserLicense() {
if (isDefined(token)) {
Server.setHttpHeader("Authorization: Bearer " + token);
Server.callWithPOST('/wp-json/wclm/v3/get-current-user-licenses', {}, function(status, response) {
Console.print(trace(response));
});
}
}
Explanation:
- Authorization Header: The JWT token is passed as a Bearer token in the authorization header.
- Endpoint: The
/wp-json/wclm/v3/get-current-user-licenses
endpoint is called to retrieve the license keys. - Callback Function: The response, which contains the user’s licenses, is printed to the console.
Step 4: Activate a License Key
The final step is to activate a specific license key. This is handled by the activateLicenseKey
function, which requires both the licenseKey
and deviceID
.
inline function activateLicenseKey(licenseKey, deviceID) {
if (isDefined(token)) {
payload = {
"license_key": licenseKey,
"device_id": deviceID
};
Server.setHttpHeader("Authorization: Bearer " + token);
Server.callWithPOST('/wp-json/wclm/v3/activate', payload, function(status, response) {
Console.print(trace(response));
});
}
}
Store the activation status and all API responses locally, cache them for future offline verifications.
Explanation:
- Payload: The payload includes the
license_key
anddevice_id
. - Endpoint: The
/wp-json/wclm/v3/activate-license
endpoint is used to activate the license key. - Callback Function: The server’s response is traced and printed to the console for debugging.
Step 5: Offline Verification
Save the last verification date locally and choose an expiration date if the user never connects to the internet.
For example, require an internet connection every 7, 15, or 30 days to ensure the user still has the license and revoke access if they don’t have it anymore.
The user opens the software:
- Is there internet access? Recheck in the background and update the last verification date.
- There is no internet access? Check if the last verification date is older than the allowed number of days of offline use.
Conclusion
By following these steps, you’ve successfully integrated the license manager API into your HISE audio plugin. This integration ensures that only users with valid licenses can access and use your plugin. You can now further customize these functions according to your specific needs or UI design.
Remember to thoroughly test these integrations to ensure they work seamlessly in your production environment. If you encounter any issues, use the console outputs to debug and refine your code. Happy coding!
More Code Snippets
Here are more useful code snippets to get you started.
Deactivate a License Key
inline function deactivateLicenseKey(licenseKey, deviceID) {
if (isDefined(token)) {
payload = {
"license_key": licenseKey,
"device_id": deviceID
};
Server.setHttpHeader("Authorization: Bearer " + token);
Server.callWithPOST('/wp-json/wclm/v3/deactivate', payload, function(status, response) {
Console.print(trace(response));
});
}
}