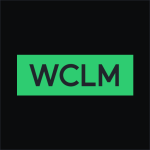
Integrating WooCommerce License Manager API v2 with Your WordPress Plugin: A Step-by-Step Guide
August 3, 2024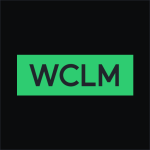
How to Automatically Complete WooCommerce Orders with License Keys: A Step-by-Step Guide
August 5, 2024Step 1: Adding a New Menu Item in the WordPress Admin
The first step in creating our license activation plugin is to add a new menu item to the WordPress admin dashboard. This menu item will link to the page where users can enter their credentials to activate their license.
// Add menu item to the WordPress admin
add_action('admin_menu', 'myplugin_license_activation_menu');
function myplugin_license_activation_menu() {
add_menu_page(
'License Activation', // Page title
'License Activation', // Menu title
'manage_options', // Capability
'license-activation', // Menu slug
'myplugin_license_activation_page' // Function to display the page content
);
}
Step 2: Displaying the License Activation Form
Next, we need to create a function that renders the form where users can enter their username and password to activate their license. This function also handles form submission and triggers the license activation process.
function myplugin_license_activation_page() {
?>
<div class="wrap">
<h1>Activate Your Product</h1>
<form method="post" action="">
<table class="form-table">
<tr valign="top">
<th scope="row">Username</th>
<td><input type="text" name="myplugin_license_username" required /></td>
</tr>
<tr valign="top">
<th scope="row">Password</th>
<td><input type="password" name="myplugin_license_password" required /></td>
</tr>
</table>
<?php submit_button('Activate'); ?>
</form>
</div>
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$username = sanitize_text_field($_POST['myplugin_license_username']);
$password = sanitize_text_field($_POST['myplugin_license_password']);
myplugin_handle_license_activation($username, $password);
}
}
Step 3: Handling License Activation
In this step, we define a function to handle the license activation process. This involves fetching a bearer token using the provided credentials, retrieving the user’s licenses, finding the relevant license, and activating it.
function myplugin_handle_license_activation($username, $password) {
$token = myplugin_get_bearer_token($username, $password);
if ($token) {
$licenses = myplugin_get_current_user_licenses($token);
$license_key = myplugin_get_relevant_license_key($licenses, '14', '20'); // Hardcoded product_id and variation_id
if ($license_key) {
$activation_response = myplugin_activate_license($token, $license_key, 'domain.ltd'); // Hardcoded device_id
myplugin_display_activation_result($activation_response);
} else {
echo '<div class="notice notice-error"><p>No valid license found for the specified product and variation.</p></div>';
}
} else {
echo '<div class="notice notice-error"><p>Invalid username or password.</p></div>';
}
}
Step 4: Fetching the Bearer Token
This function is responsible for obtaining a bearer token by sending the provided username and password to the authentication endpoint.
function myplugin_get_bearer_token($username, $password) {
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://demo.firassaidi.com/wc-license-manager/wp-json/jwt-auth/v1/token',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => http_build_query(array('username' => $username, 'password' => $password)),
));
$response = curl_exec($curl);
curl_close($curl);
$data = json_decode($response, true);
return isset($data['token']) ? $data['token'] : false;
}
Step 5: Retrieving User Licenses
With the bearer token, we can now fetch the licenses associated with the authenticated user. This function sends a request to the appropriate endpoint to get the user’s licenses.
function myplugin_get_current_user_licenses($token) {
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://demo.firassaidi.com/wc-license-manager/wp-json/wclm/v3/get-current-user-licenses',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer ' . $token,
),
));
$response = curl_exec($curl);
curl_close($curl);
return json_decode($response, true);
}
Step 6: Finding the Relevant License Key
After retrieving the licenses, we need to find the relevant license key based on the hardcoded product ID and variation ID. This function iterates through the licenses to find the matching one.
function myplugin_get_relevant_license_key($licenses, $product_id, $variation_id) {
if (!empty($licenses['response']['licenses'])) {
foreach ($licenses['response']['licenses'] as $license) {
if ($license['product_id'] == $product_id && $license['variation_id'] == $variation_id) {
return $license['license_key'];
}
}
}
return false;
}
Step 7: Activating the License
With the relevant license key, we can now activate the license by sending a request to the activation endpoint. This function handles the activation process and returns the response.
function myplugin_activate_license($token, $license_key, $device_id) {
$curl = curl_init();
$parameters = array(
'license_key' => $license_key,
'device_id' => $device_id
);
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://demo.firassaidi.com/wc-license-manager/wp-json/wclm/v3/activate',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => http_build_query($parameters),
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer ' . $token,
),
));
$response = curl_exec($curl);
curl_close($curl);
return json_decode($response, true);
}
Step 8: Displaying Activation Result and Redirecting
Finally, we need to display the result of the activation process and redirect the user to a different admin page if the activation is successful. This function handles that logic.
function myplugin_display_activation_result($response) {
if ($response['response']['result'] == 'success') {
update_option('myplugin_is_active_option', true);
wp_redirect(admin_url('admin.php?page=myplugin-license-activated'));
exit;
} else {
update_option('myplugin_is_active_option', false);
echo '<div class="notice notice-error"><p>' . esc_html($response['response']['message']) . '</p></div>';
}
}
Step 9: Creating the Success Page
We need to create a hidden page in the admin dashboard to display a success message after successful activation. This function adds a submenu page that can be accessed directly via URL.
// Add menu item for the activation success page
add_action('admin_menu', 'myplugin_license_activation_success_menu');
function myplugin_license_activation_success_menu() {
add_submenu_page(
null, // No parent menu
'License Activated', // Page title
'License Activated', // Menu title
'manage_options', // Capability
'myplugin-license-activated', // Menu slug
'myplugin_license_activation_success_page' // Function to display the page content
);
}
By following these steps, you can create a WordPress plugin that allows users to activate products using license keys. Each function is designed to handle a specific part of the process, from adding a menu item to handling form submission, retrieving licenses, and displaying the activation result. This modular approach ensures that the plugin is easy to understand and maintain.